Looping is a fundamental concept in all programming languages, not just Python. They are used to repeat a block of code multiple times as per our requirement.
There are mainly two types of loops in Python. They are :
- for loop
- while Loop
In this tutorial we are going to focus on the while
loop.
The while
loop in Python is used to iterate over a block of code as long as the test condition evaluates to True
.
Syntax of while Loop in Python
The syntax of the while
loop in Python is:
while test_condition:
statement(s)
Here, the statements inside the while
loop are executed for as long as test_condition
is True
.
At first, test_condition
is checked and if it is True
then the body of the loop is entered. After one iteration, the test_condition
is checked again. And this process continues until test_condition
evaluates to False
.
Flowchart of while Loop
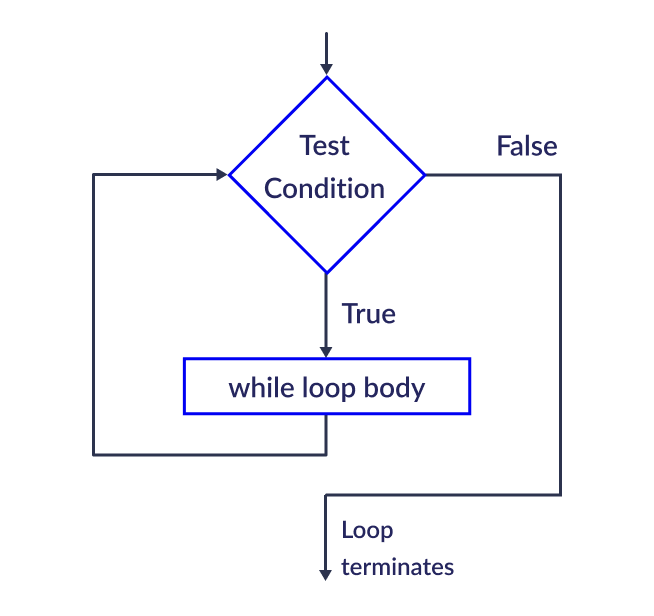
Example 1: Python while Loop
print('Number from 1 to 5:')
n = 1
while n <= 5:
print(n)
n = n + 1
Output
Number from 1 to 5: 1 2 3 4 5
Here is how this program works.
Iteration | Variable | Condition: n | Action |
---|---|---|---|
1st | n = 1 (initialized) |
true |
1 is printed.n is increased to 2. |
2nd | n = 2 |
true |
2 is printed.n is increased to 3. |
3rd | n = 3 |
true |
3 is printed.n is increased to 4. |
4th | n = 4 |
true |
4 is printed.n is increased to 5. |
5th | n = 5 |
true |
5 is printed.n is increased to 6. |
6th | n = 6 |
false |
The loop is terminated. |
Example 2: Python while Loop
sum = 0
# Take user input
n = int(input('Enter a number: '))
# Loop until n is not equal to 0
while n != 0:
# add the value of n to sum
sum = sum + n
# take the input again
n = int(input('Enter a number: '))
# print the sum
print('Result :', sum)
Output
Enter a number: 3 Enter a number: 1 Enter a number: 1 Enter a number: 0 Result : 5
Here is how this program works.
Iteration | Variables | Condition: n != 0 | Action |
---|---|---|---|
1st | sum = 0 n = 3 |
(3! = 0) i.e. True |
sum = 0 + 3 Enter a number is printed |
2nd | sum = 3 n = 1 |
(1! = 0) i.e. True |
sum = 3 + 1 Enter a number is printed |
3rd | sum = 4 n = 1 |
(1! = 0) i.e. True |
sum = 4 + 1 Enter a number is printed |
4th | sum = 5 n = 0 |
(0! = 0) i.e. False |
The loop is terminated.Result : 5 is printed |
Infinite while Loop
If the condition of a while
loop is always True
, the loop runs for infinite times (until the memory is full). This is called infinite while
loop. For example,
while (True):
print('infinite Loop')
Output
infinite Loop infinite Loop infinite Loop . . .
Recommended Reading: Python for loop