In programming, a variable is a container that is used to store values. For example,
age = 25
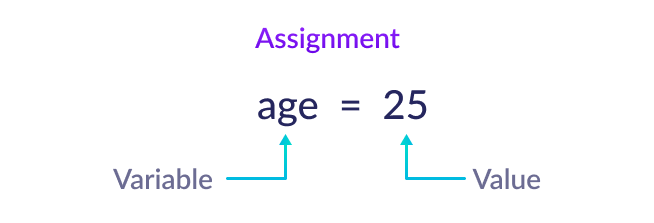
Here,
- age is a variable,
- 25 is the value stored in the variable age, and
=
is the operator used to store value to a variable.
Variables can store different types of data types. In this example, the variable age is storing an integer type.
To learn more about data types, visit Python data types.
Print Variables in Python
We can simply print a variable using the print() function. For example,
age = 25
# print the variable age
print(age) # 25
Note that if we use the quotation ''
in the print statement, Python considers it as a string. For
example,
age = 25
# variable with quotation
# prints the string
print('age')
# Output: age
Declare Variables Before Use
We cannot use a variable before defining them. For example,
age = 25
# use the name variable without defining it
# error
print(name)
print(age)
Output
NameError: name 'name' is not defined
Change Value of a Variable
In Python, we can change the value stored in a variable. For example,
city = 'New York'
print(city) # New York
# change the value of city
city = 'California'
print(city) # California
Here, the value of the variable city is changed from 'New York'
to 'California'
.
Note: You do not have to explicitly define the variable type in Python. When we
assign 'New York'
to the variable city, it automatically declares city as a
string.
Assign One Variable To Another
We can also assign one variable to another. For example,
city = 'New York'
destination_city = 'California'
# assign one variable to another
city = destination_city
print(city) # California
Here, we have assigned the value of destination_city to city using the =
operator.
Rules of Naming Variables in Python
Here are the rules for naming variables:
- A variable name can consist of alphabets, digits, and an underscore.
- Variables cannot have special symbols like $, @, #, etc.
- Variable names cannot begin with a number. For example, 1name.
By the way, we should always try to give meaningful variable names. This makes your code easier to read and understand.
Good, Bad and Illegal Variable Names
Illegal Variable | Bad Variable | Good Variable |
---|---|---|
date time | dati | dateTime |
city-name | cn | city_name |
20n | numb | number |
s@lary | sal | salary |